Rapid Prototyping with Ionic 2 and Node.js - Part 1 Last update: 2017-01-10
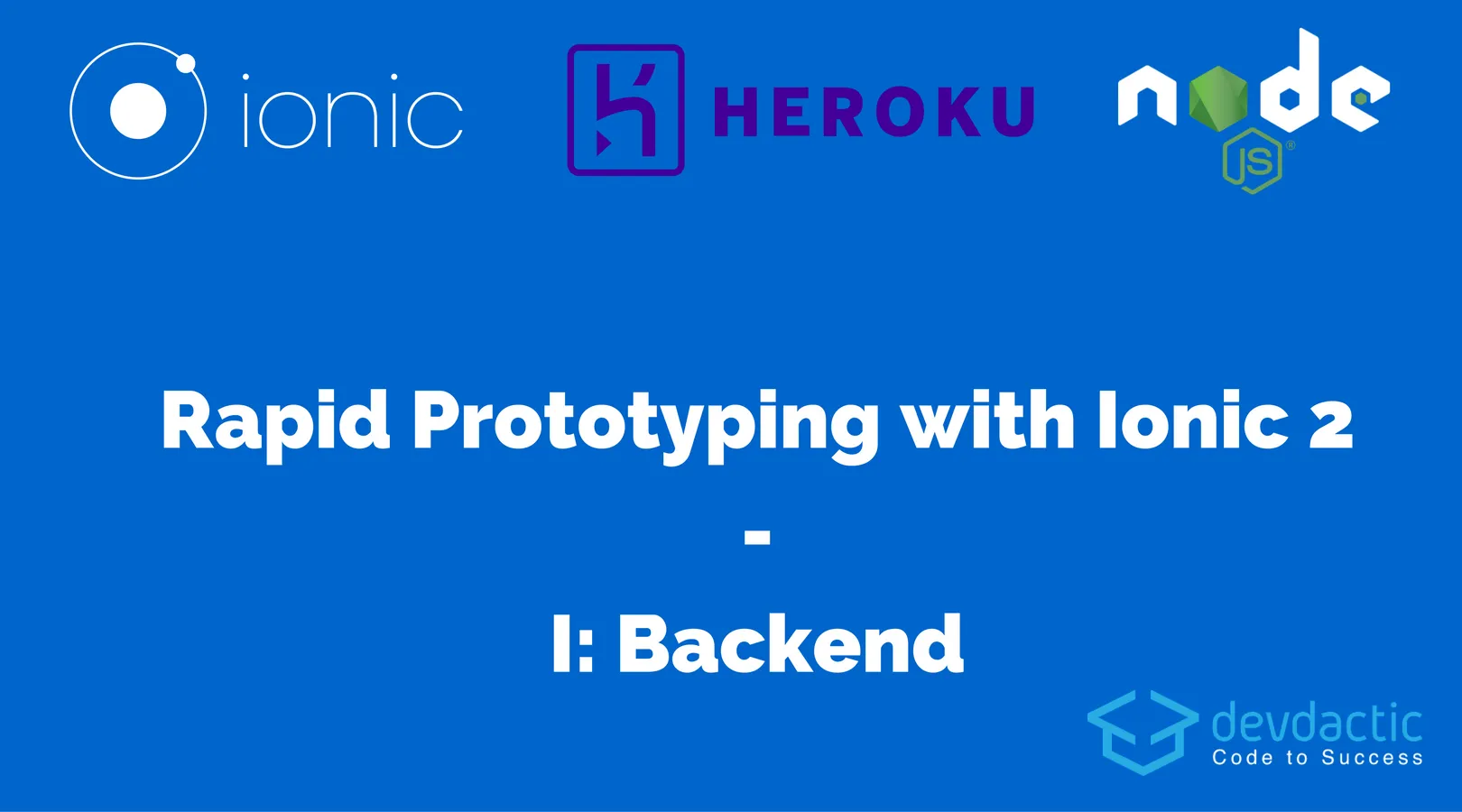
Do you know how fast you can actually develop a really solid MVP prototype including a frontend and working backend these days? It’s amazing.
In this 3 part series I will share how I recently assembled a fully working prototype in only 3 days using node.js as the backend and Ionic 2 as the frontend of our MVP.
Prerequisite
Learning Ionic can become overwhelming, I know that feeling. Is learning from tutorials and videos sometimes not enough for you? Then I got something for you.
If you want to learn Ionic with step-by-step video courses, hands-on training projects and a helpful community who has your back, then take a look at the Ionic Academy.
The Plan
The 3 parts of the series can be found below:
Rapid Prototyping with Ionic 2 and Node.js - Part 1: The Node.js Backend Rapid Prototyping with Ionic 2 and Node.js - Part 2: The Ionic 2 Frontend Rapid Prototyping with Ionic 2 and Node.js - Part 3: Deployment of your Components
If you want to stay up to date and get noticed once the other articles are out, make sure to leave your email in the box below!
In the first part we will start with a simple Node.js backend including a MongoDB database for holding some information.
Inside the second part we develop the Ionic 2 app and connect to our backend to retrieve the data.
As all of this is only working locally, we need a way to get this out in the hands of friends and customers so inside the third part we will take care of the deployment and actually getting our work into the hands of others!
If you got any questions along the way simply leave a comment. Let’s get the fun started!
Setting up Node and MongoDB Server
First of all make sure you have Node.js installed on your machine. Additional we will use a MongoDB to hold our data, so go ahead and install it as well following the instructions.
To test our app later I always recommend to use the Postman Extension which makes it really easy to test any REST API.
Finally, to inspect your MongoDB it’s convenient to have some GUI for the database so I picked Robomongo for that!
You don’t need all of these tools, but of course you need Node.js and MongoDB created to actually run our server.
Starting a new Node.js Server
To start a new Node.js server you have to run only one command which will first of all create your package.json. Afterwards we install the needed npm packages and finally we add 4 files that will be filled with everything we need later.
While the npm creation runs you will get asked many questions. Most of them can be skipped by pressing enter using the default value, but make sure to set the entry point of your app to server.js. Of course you can change it later inside the package.json, but why not do it right in the first place here.
Now go ahead and run:
npm init
npm install --save morgan cors express errorhandler mongoose body-parser helmet
touch server.js
touch todo.js
touch todo-routes.js
touch config.json
We are now ready to fill all these beautiful files with some great code!
Creating the Database Model using Mongoose
In order to interact with our database we use the Mongoose npm package. The way to use the database is to have a scheme defined that represents documents in the database.
In our case, we define a simple TodoSchema which represents a todo with a text and date.
Additional we add a pre function that will always be executed before the object is saved to the database. Here we can change things, like in our case set the created_at
variable to the current date.
Finally we export that schema so the rest of our app can actually use it. Now go ahead and add the following to the todo.js:
var mongoose = require('mongoose');
var Schema = mongoose.Schema;
var TodoSchema = new Schema({
text: {
type: String,
required: true
},
created_at: Date,
});
TodoSchema.pre('save', function (next) {
var todo = this;
// get the current date
var currentDate = new Date();
// if created_at doesn't exist, add to that field
if (!todo.created_at) {
todo.created_at = currentDate;
}
next();
});
module.exports = mongoose.model('Todo', TodoSchema);
We also created a config file which holds the url to our database. Currently we only use the localhost, but this file will become more useful in the 3. part of the series.
For now, open the config.json and insert:
{
"database": "mongodb://localhost/devdactic-rapidbackend"
}
Make sure to start your MongoDB (on Mac simply run mongod
from the CL) in order to get a connection from our app to the database!
Adding Routes with Express
The data model is finished, let’s continue with the actual REST routes for our app.
In our case, we will define 3 different routes:
- GET todos: Get a list of all todos in the database
- POST todos: Create a new todo and save to the database
- DELETE todos/:todoId: Delete a specific todo from the database by its ID
We could have these routes inside the server file, but to keep a clean structure we put them in an appropriate place, which is in our case the todo-routes.js so go ahead and insert:
var express = require('express');
var app = module.exports = express.Router();
var Todo = require('./todo');
// POST
// Create a new Todo
app.post('/todos', function (req, res) {
if (!req.body.text) {
return res.status(400).send({ "success": false, "msg": "You need to send the text of the todo!" });
}
var newTodo = new Todo({
text: req.body.text
});
newTodo.save(function (err) {
if (err) {
console.log("some error: ", err);
return res.json({ "success": false, "msg": "Error while creating Todo", "error": err });
}
res.status(201).send({ "success": true, "msg": 'Successful created new Todo.' });
});
});
// GET
// Get all open Todos
app.get('/todos', function (req, res) {
Todo.find({}, function (err, todos) {
if (err) {
return res.json({ "success": false, "msg": "Error while creating Todo", "error": err });
}
res.status(200).send({ "success": true, "result": todos });
});
});
// DELETE
// Remove one todo by its ID
app.delete('/todos/:todoId', function (req, res) {
var lectionId = req.params.todoId;
if (!lectionId || lectionId === "") {
return res.json({ "success": false, "msg": "You need to send the ID of the Todo", "error": err });
}
Todo.findByIdAndRemove(lectionId, function (err, removed) {
if (err) {
return res.json({ "success": false, "msg": "Error while deleting Todo", "error": err });
}
res.status(200).json({ "success": true, "msg": "Todo deleted" });
});
});
To create a new todo, we create a new Todo
object which is our mongoose scheme that we have exported. This object already has different functions, like save
to write the object to the database. If this operations is successful we return a success and are finished here.
To get all todos we can use the Todo
schema again and call find
without any specific parameters. Normally you could specify an object to filter here, but in our case we want all the todos inside the database. The return and error handling is pretty much the same in all of our 3 routes.
Finally, to delete a todo we get an id through the request parameters which we use then for the findByIdAndRemove
function of our TodoSchema.
That’s already everything for some very simple CRUD (well not the U but..) operations on our MongoDB, now we only need to get this server started!
Starting our Node.js Server
We have created the model and the routes, but we actually need to define our server and apply some of our installed packages to make life easier.
Stuff like helmet (protects your app) and cors (you know about CORS with Ionic apps) are just really helpful and can be added within a few lines.
Finally, we establish the Mongoose connection to the database using our config.json and call use
on our todo-routes so the app applies all the routes to our server. Now we can create the server and print out a log if everything was successful.
Go ahead and add everything to your server.js:
var logger = require('morgan'),
cors = require('cors'),
http = require('http'),
express = require('express'),
errorhandler = require('errorhandler'),
bodyParser = require('body-parser'),
mongoose = require('mongoose'),
helmet = require('helmet'),
config = require('./config.json');
var app = express();
app.use(helmet())
app.use(bodyParser.urlencoded({ extended: true }));
app.use(bodyParser.json());
app.use(cors());
if (process.env.NODE_ENV === 'development') {
app.use(logger('dev'));
app.use(errorhandler())
}
var port = process.env.PORT || 3001;
mongoose.Promise = global.Promise;
mongoose.connect(config.database);
app.use(require('./todo-routes'));
http.createServer(app).listen(port, function (err) {
console.log('listening in http://localhost:' + port);
});
If your package.json has the correct entry point you can start your server now simply by running npm start
!
You can see a detailed video including how to use Postman and Robomongo below.
sionThe development of a very basic REST API using Node.js, Express and MongoDB is extremely fast and is a solid approach to rapid prototyping. The developed assets can build the base for the future project and can be expanded to a full-blown backend over time, so it’s not a quick shot for a prototype and starting over again but a very reasonable start for a modern backend API project.
Happy Coding, Simon