10 Common Ionic Problems & Error Messages (And How to Fix Them) Last update: 2018-01-16
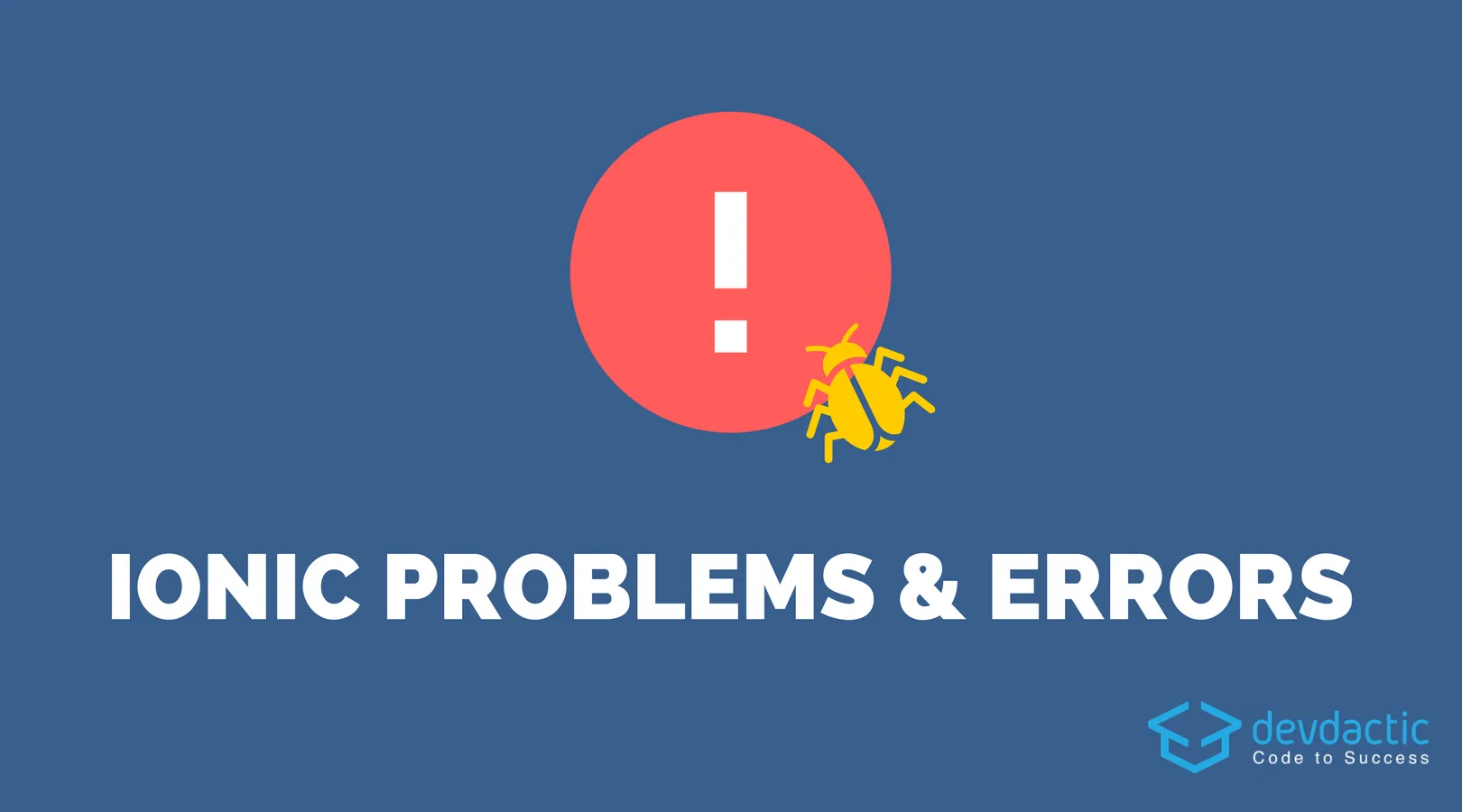
We have all seen many error messages while developing our Ionic apps. Some appear more frequent, others appear only on very special occasions. But especially for beginners these messages can be challenging as the meaning of Ionic Problems is not always clear.
The problem most of the time is that your app will run fine and the build is successful - the problems only occur at runtime and therefore you are completely dependent on the hin inside the error message and understanding those messages is an essential part of being a good developer.
In this article I’ll outline 10 common error messages and problems that can happen quite often and how each of them can be easily solved within your Ionic app!
1. Cannot read property ‘xyz’ of undefined
A very classic error and one that can be easily tracked down although it’s not immediately clear where to look for the solution. This error comes up when you have code like this:
// Inside your class
item: string;
// Inside your view
{{ item.myValue }}
The property of your object is not the problem but the object itself; you are trying to access a property of an element that’s undefined (or in other cases null).
Check where you initialise your object or use the elvis operator to access properties safely without breaking your apps code:
// ? operator prevents crashing here!
{{ item?.myValue }}
2. Uncaught (in promise): invalid link: MyPage
This error appears when you try to navigate to a page that’s not existing. Perhaps you spelled the name wrong?
Especially when using lazy loading and passing page names as string, it can be tough to spot the problem as the linting won’t give you any indication that something is wrong.
Also when you get a message like ”Nav Link error MyPage” it’s related to the same obstacle, some navigation is simply not working because Ionic can’t find the page you want to navigate to (or which you have used as root for e.g. a Tab bar).
As a solution, search your project for the name of the page of the error message and see if it’s actually the real name of the page you want to display!
3. _co.myFunction is not a function
This error is quite easy to find, it indicates you are calling a function from your template which you haven’t implemented inside your class.
To fix this, simply make sure that you have a function declared with the according name and that everything is spelled correctly. Again, this error won’t come up through linting because JavaScript won’t find errors within your template that are related to the class. Perhaps one day we get this..
4. Cannot find control with name: ‘myName’
If you are using reactive forms there are all kind of errors that can occur. This one is a very basic one and it indicates you are simply trying to use a formControl
which you haven’t defined.
// Inside your class
this.registrationForm = this.fb.group({
username: ['Simon', [Validators.required]]
});
// Inside your view
<form [formGroup]="registrationForm">
<ion-input type="text" formControlName="myName"></ion-input>
</form>
In the code above you can see that the form has a username control, but the name used inside the view is myName which is not part of the definition!
5. Type TestPage is part of the declarations of 2 modules
Now things get a bit more tricky, as this error can be very hard to understand for beginners. What actually is a module?
When you use lazy loading for your pages, new generated pages will have their own module file right next to them. Without lazy loading, pages don’t have their own module file.
The trick is: If you page doesn’t have a module file (which means you are not using lazy loading) you need to reference all your pages inside your app.module.ts file.
But if the page already has it’s module and you import it somewhere else (for whatever reason) this error will come up and tell you that you can’t have the page in 2 modules.
Therefore, check if you import your page in some place where it shouldn’t be and make sure it’s only imported in one module file!
6. No Provider for xyz
You think you are pro structuring your code and outsourcing your business logic to a provider, you inject it through your constructor but ffs why does this error appear?
This message is not really indicating the fix very good and therefore can be hard to find. The problem is, you need to let Angular know about the providers of your app so the internal Injector can actually put the right provider into your class.
This error is related to dependency injection and there’s a big internal process behind how you can get your providers through your constructor.
The fix?
You need to add your created Provider to the array of providers inside the app.module.ts so your app knows about it. With newer releases of the Ionic CLI this step should automatically happen, but in case it’s not you know how to fix it now.
7. Cannot find a differ supporting object ’[object Object]’ of type ‘object’
Working with arrays inside your view and using *ngFor
is an awesome feature of Angular, but you can easily break things if you don’t watch out. The error message is not super helpful, but it has some additional information most of the time:
”Only arrays and iterables are allowed Error: Cannot find a differ supporting object ’[object Object]’ of type ‘object’. NgFor only supports binding to Iterables such as Arrays.”
This should make more sense, but let’s add a code example.
// Inside your class
items = {};
// Inside your view
<ion-list>
<ion-item *ngFor="let foo of items"></ion-item>
</ion-list>
The problem in the example above is easy to spot: You are trying to iterate over an object, but ngFor expects an array!
While you can get all keys of an object, it is not meant to be used this way.
Most of the time this error appears when you get data from a REST API and you are not sure about the values. Perhaps you think something is an array, but it actually isn’t.
So always check your responses, pick the right key where the result contains an array and make sure you are not passing a plain object to the ngFor!
8. Template parse errors: ‘custom-component’ is not a known element
Now we got 2 errors that will immediately break your app once it starts, but the problem won’t appear before (so build is still successful).
You can already note that Template parse errors means there’s a problem within your template, your view file.
To get this error you might have something like this in your view:
<ion-content>
<special-component></special-component>
</ion-content>
You have created a Component and want to use it within your view. The problem is, by default the Ionic generated components are only added to a ComponentsModule
, and you have to import this module where you need it!
This means, you can either import it at the top level of your app in app.module.ts or with lazy loading import the module of your components only in the module file of your pages.
Then Angular will know how to reference this special tag of your view and insert the correct component that you’ve created before.
9. Template parse errors: Can’t bind to ‘enabled’ since it isn’t a known property of ‘button’.
Another error that can occur with tags is this one, and it’s actually pretty easy to track down.
Imagine a code like this:
<button ion-button [enabled]="false">Test</button>
Seem fine from the outside, but the problem is that enabled
is not a property of button you can bind to!
A fix for this is to use:
<button ion-button [disabled]="false">Test</button>
This is just one example, in general it simply means that you try to bind to something on the tag that’s not really available. It cna happen for all tags, your components and all the things you use in your view.
If you are unsure about the properties of elements, simply check out the according API documentation and see what’s available!
10. cordova_not_available
To wrap this list up, let’s end with a super classic one that hopefully everyone already knows about.
If you code your app and use the live preview within your browser, most of the time all is fine. But once you start to integrate Cordova plugins, you can’t test or use those features within the simple preview.
The Cordova.js is only injected once you build your app on a device (or simulator), and therefore none of those features will work inside the preview and you’ll run into this error.
The fix is simple: Just run your app on a device or, a bit more advanced way, is to mock your plugins.
You can override the Plugins so even on the browser the call returns a dummy result and not an error. If you don’t want to write all your Mocks on your own, check out this awesome package from Chris Griffith to get a full set of Mock objects!
11. BONUS: Nothing works?
Every good list needs a little bonus… And this one is rather simple.
Sometimes you fix all the problems, you are sure that everything is fine and still nothing works or nothing changes.
The solution?
Restart your livereload. Trust me, it has helped me and my students hundreds of times.
From time to time, Ionic releases can contain a few minor issues that break stuff. Things that worked don’t work like that should in the next release. It can happen.
Especially if you generate new files with the CLI I encountered this problem often, and the fix was just to close the command and start it again.
If you are lucky, everything will just work again then!
Anything missing?
Do you have any more common issues you’ve stumbled upon while developing your next epic Ionic app?
Let us know in the comments below and I’ll happily add them to the list to cover even more typical Ionic and Angular error messages!