Swipeable Cards with the Ionic Framework Last update: 2015-03-20
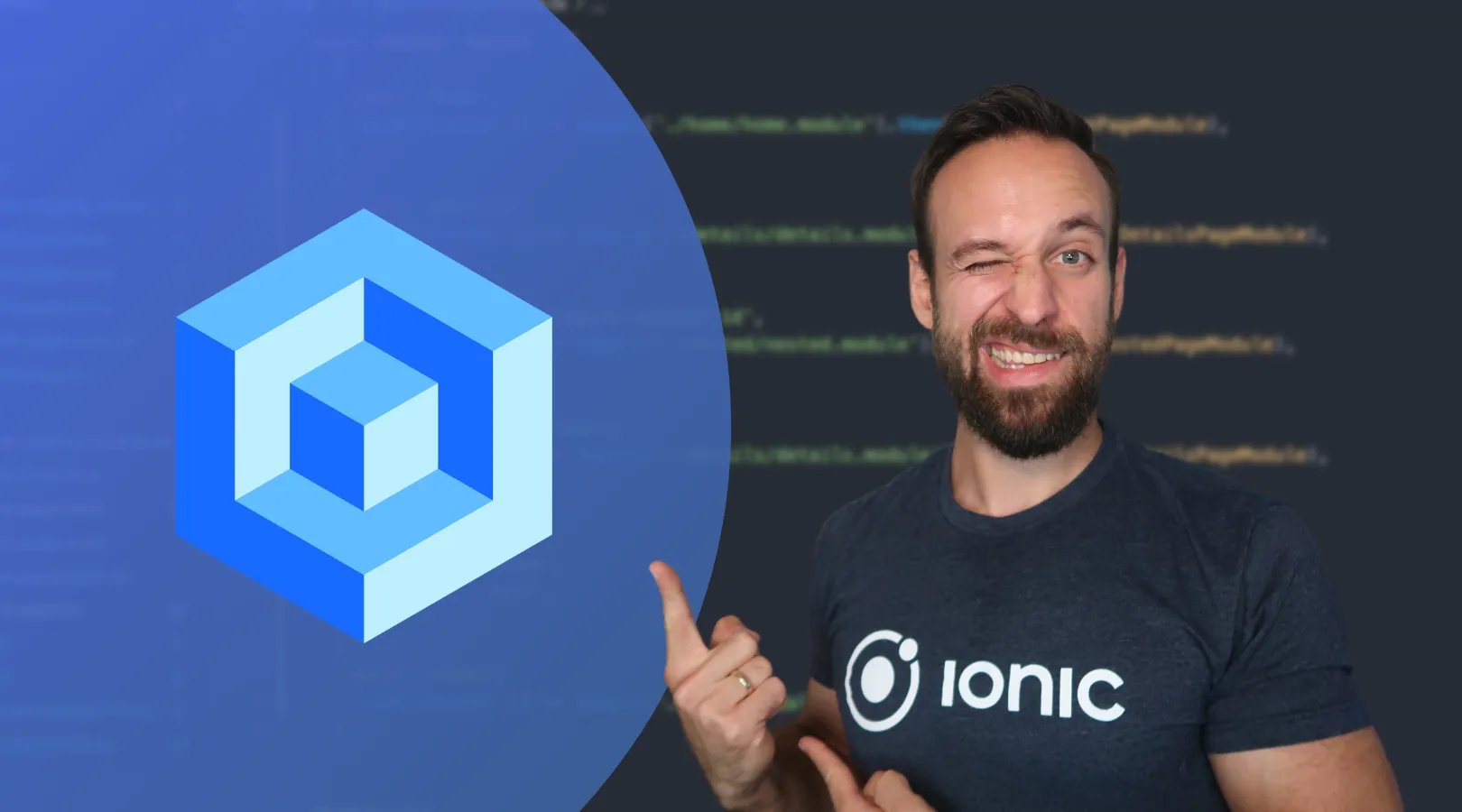
Swipeable cards are nowadays used very often in mobile applications as a feature to navigate through a list of items/images. In a recent tutorial I explained how to createTinder Style Cards with Ionic. This time, I will show you how to get swipeable cards like seen in the jelly app.
To get this neat feature we will use the swipe cards ion from Ionic.
Setup a demo project
We start with a blank Ionic project and install the custom ion with bower:
ionic start devdactic-swipeable blank
cd devdactic-swipeable
bower install ionic-ion-swipe-cards --save
After installing the ion, we need to add the dependency to our angular.module
array of dependencies inside app.js:
angular.module('starter', ['ionic', 'ionic.contrib.ui.cards'])
Additional we have to load the installed script inside our index.html after app.js:
<script src="lib/ionic-ion-swipe-cards/ionic.swipecards.js"></script>
Now we are ready to add some cool functions with this ion!
Creating the view for swipeable cards
First of all, we add everything for our view inside our index.html inside the body. We need to apply a controller to our view, then we add the custom ion with some elements inside it.
<ion-pane ng-controller="CardsCtrl">
<ion-header-bar class="bar-stable">
<h1 class="title">Ionic Blank Starter</h1>
</ion-header-bar>
<swipe-cards>
<swipe-card on-card-swipe="cardSwiped()" id="start-card">
Swipe down for a new card
</swipe-card>
<swipe-card
ng-repeat="card in cards"
on-destroy="cardDestroyed($index)"
on-card-swipe="cardSwiped($index)"
>
<div ng-controller="CardCtrl">
<div class="title">{{card.title}}</div>
<div class="image">
<img ng-src="{{card.image}}" />
</div>
<div class="button-bar">
<button class="button button-clear button-positive" ng-click="doAnything()">Like</button>
<button class="button button-clear button-positive" ng-click="doAnything()">
Disklike
</button>
</div>
</div>
</swipe-card>
</swipe-cards>
</ion-pane>
The first swipe-card is just a start card which will lay in the background of our view. In general we create a swipe-card for every card in cards
which we will fill from our controller. The swipe card directive offers the opportunity to catch the on-swipe
and on-destroy
events, so we catch those and handle them in our controller.
Inside the card we have an additional controller, which is optional. If you want to have buttons or some specific actions on your cards, you will need one. The card finally consists of a title, an image and two buttons which call an action from the CardCtrl
.
Creating the controllers for swipeable cards
For our swipeable cards we need 2 controllers, the CardsCtrl
for the general view and the CardCtrl
which is assigned to every single card.
To get some cards, we will use the randomuserapi and grab some data for dummy mails and images. Our array will consist of one card in the beginning. Whenever we swipe out a card, we will add a new one, and when the card is finally destroyed we remove it from our cards array. So go ahead and add the first controller to your app.js:
.controller('CardsCtrl', function($scope, $http, $ionicSwipeCardDelegate) {
$scope.cards = [];
$scope.addCard = function(img, name) {
var newCard = {image: img, title: name};
newCard.id = Math.random();
$scope.cards.unshift(angular.extend({}, newCard));
};
$scope.addCards = function(count) {
$http.get('http://api.randomuser.me/?results=' + count).then(function(value) {
angular.forEach(value.data.results, function (v) {
$scope.addCard(v.user.picture.medium, v.user.email);
});
$scope.showCards = true;
});
};
$scope.addCards(1);
$scope.cardSwiped = function(index) {
$scope.addCards(1);
};
$scope.cardDestroyed = function(index) {
$scope.cards.splice(index, 1);
};
});
The second controller is just for handling the button event we created inside our view, so add this one below the just created controller:
.controller('CardCtrl', function($scope, $ionicSwipeCardDelegate) {
$scope.doAnything = function() {
var card = $ionicSwipeCardDelegate.getSwipeableCard($scope);
card.swipe();
};
});
When we tap the button, we call the injected $ionicSwipeCardDelegate
to grab the current card. On this card we can now call swipe()
which shows a way of programmatically swiping out the cards! The controllers are fine now, the last thing open is to add a bit cooler UI.
Adding custom CSS for a cool look
The current look is not really what we would like to have. To get the expected result, we need to change a few lines inside our style.css. The code is in most parts just copied from the Ionic official example, so cheers to the guys @Ionicframework for their awesome work!
.pane {
background-color: #333;
}
.swipe-cards {
position: fixed;
}
swipe-card {
display: none;
position: fixed;
-webkit-transform: scale(1, 1);
left: 50%;
top: 50%;
width: 300px;
height: 300px;
margin-left: -150px;
margin-top: -150px;
box-sizing: border-box;
background-color: rgb(255, 255, 255);
border-radius: 4px;
overflow: hidden;
-webkit-animation-fill-mode: forwards;
}
swipe-card .title {
height: 40px;
padding: 5px;
line-height: 30px;
color: #444;
}
swipe-card .image {
overflow: hidden;
max-height: 210px;
}
swipe-card .button {
border: none;
}
swipe-card .image img {
width: 100%;
border-radius: 0px 0px 4px 4px;
}
#start-card {
color: #fff;
background-color: #30bd8a;
line-height: 300px;
word-wrap: break-word;
border: 6px solid #4cd68e;
text-align: center;
}
#start-card span {
display: inline-block;
line-height: 40px;
width: 200px;
font-size: 30px;
vertical-align: middle;
}
@-webkit-keyframes bounceIn {
0% {
-webkit-transform: scale(0, 0);
}
70% {
-webkit-transform: scale(1.2, 1.2);
}
100% {
-webkit-transform: scale(1, 1);
}
}
swipe-card.pop-in-start {
-webkit-transform: scale(0, 0);
}
swipe-card.pop-in {
-webkit-animation: bounceIn 0.4s ease-out;
}
As you can see there is a lot of styling included here, but the result is great! Run your app inside the browser or on a device and get your hands on the nice effect of swipeable cards, it should a lot like this:
Conclusion
This tutorial explained how to use a custom ion to get a swipeable cards effect inside your Ionic app. The code for the view and the controllers is very straight forward, only the CSS is a bit too much in my eyes, maybe they will shift some of that to the general directive of swipe cards in the future.
See a video version of this article below.
If this tutorial was helpful, follow me on twitter @schlimmson and leave a comment/ tweet it! Don’t forget to subscribe to my newsletter to receive new posts and info via mail!
So long, Saimon