How to Deploy Ionic Apps as Website to Heroku Last update: 2017-10-31
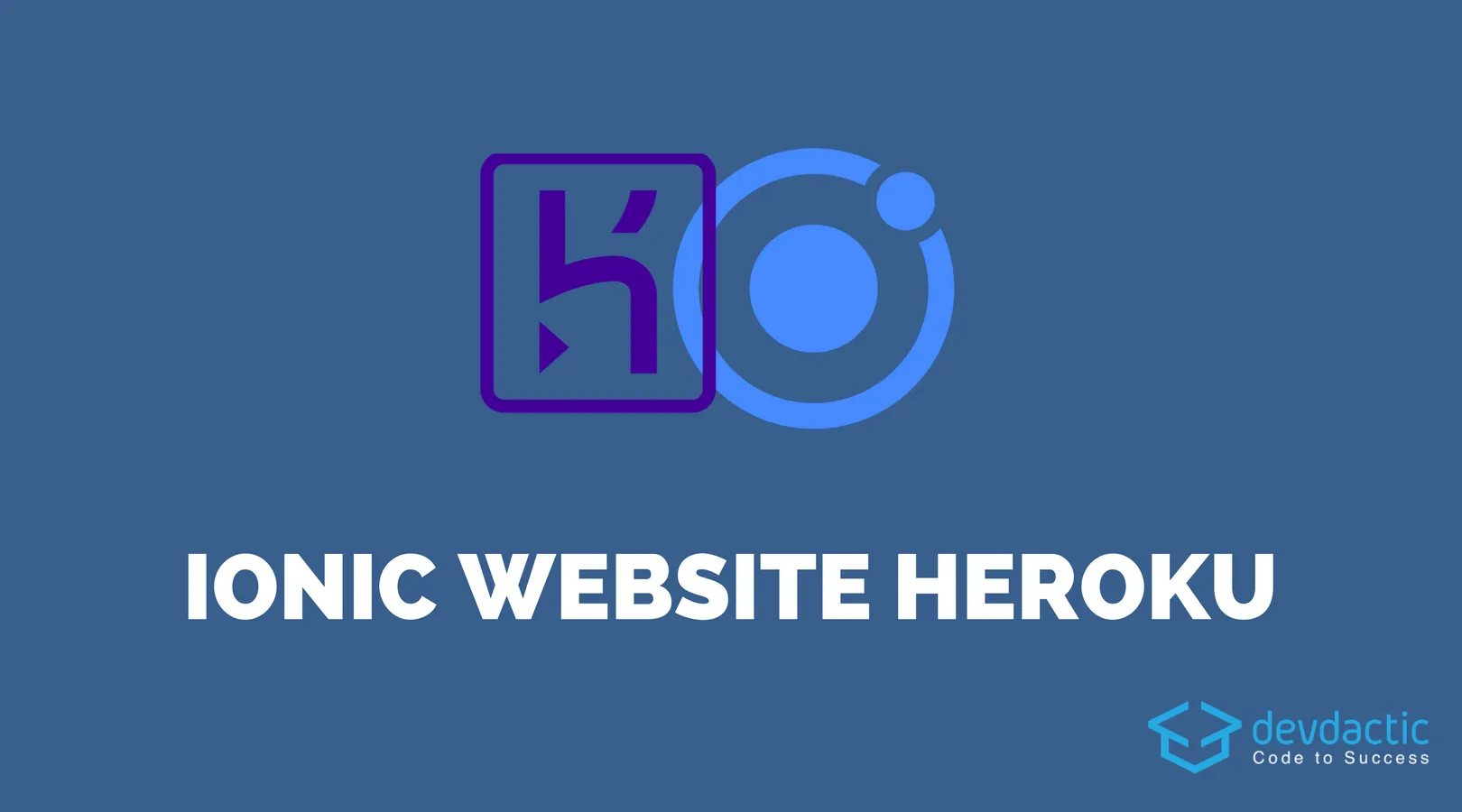
Our Ionic App is in itself a simple website, and if you want to build your project for mobile and as a webpage, there’s an easy way to make your app available to the whole world with Heroku which allows to deploy Ionic apps (and all other web contents!).
Heroku is a platform to easily deploy your application in a container without thinking about much else. We just need to find a way to bring our Ionic app to Heroku in the correct format so Heroku can take care of the rest!
Before starting, make sure you have a Heroku account and also install the Heroku Toolbelt!
Starting our Ionic App
We start with a blank Ionic app, but of course you can also just change your existing app. Go ahead and run:
ionic start herokuApp blank
cd ./herokuApp
npm install --save morgan cors express body-parser
As you can see we not only start a new app but install a few dependencies you might know from NodeJS server development!
That’s because inside Heroku we will start a super simple NodeJS server which will host the files for our Ionic app. Therefore, go ahead and create this server.js at the top-level of your Ionic project and insert:
var express = require('express');
var app = express();
var morgan = require('morgan');
var bodyParser = require('body-parser');
var cors = require('cors');
app.use(morgan('dev'));
app.use(bodyParser.urlencoded({'extended':'true'}));
app.use(bodyParser.json());
app.use(cors());
app.use(function(req, res, next) {
res.header("Access-Control-Allow-Origin", "*");
res.header('Access-Control-Allow-Methods', 'DELETE, PUT');
res.header("Access-Control-Allow-Headers", "Origin, X-Requested-With, Content-Type, Accept");
next();
});
app.use(express.static('www'));
app.set('port', process.env.PORT || 5000);
app.listen(app.get('port'), function () {
console.log('Express server listening on port ' + app.get('port'));
});
It’s basically some general settings for CORS and other stuff, but the most important part is that the app will use our www/ folder which holds the build of our Ionic app!
Before we now get into the deployment, let’s make a small change to display a pop-up with the current platform we are on.
This can be really helpful to see if your app can use Cordova plugins or not, because deployed as a website you won’t have access to these plugins! Therefore, we add a button to our src/pages/home/home.html like this:
<ion-header>
<ion-navbar>
<ion-title>
Ionic Web
</ion-title>
</ion-navbar>
</ion-header>
<ion-content padding>
<button ion-button full (click)="showPlatform()">Where am I running?</button>
</ion-content>
The function that will be called will get the current platforms we are running on and display them inside an Alert, so open your src/pages/home/home.ts and change it to:
import { Component } from '@angular/core';
import { NavController, AlertController, Platform } from 'ionic-angular';
@Component({
selector: 'page-home',
templateUrl: 'home.html'
})
export class HomePage {
constructor(public navCtrl: NavController, private alertCtrl: AlertController, private platform: Platform) {
}
showPlatform() {
let text = 'I run on: ' + this.platform.platforms();
let alert = this.alertCtrl.create({
title: 'My Home',
subTitle: text,
buttons: ['Ok']
});
alert.present();
}
}
To create our new Heroku App we can use our toolbelt, so simply run:
heroku login
# Do the login stuff
heroku create
Alright we are ready, but now we got a little problem:
Normally, our Ionic app is built using the installed Ionic CLI and app scripts on your environment, but those are not (automatically) available on Heroku.
This means, we need to find a way to either bring the locally built folder to Heroku or to enable Heroku to build our Ionic app on its own. For this tutorial I’ll explain the second approach, because the first one involves to comit your build folder to Git which is not recommendable.
Add Ionic Scripts as Dependency
The first step is to move 2 devDependencies a bit up to the real dependencies. Therefore, change your package.json so it looks like this:
"dependencies": {
...
"@ionic/app-scripts": "2.1.4",
"typescript": "2.3.4"
},
"devDependencies": {
},
Now the dependency to the build scripts will also be installed once we deploy the app, and we just need to tell Heroku to run this build step before starting our app.
For this we can use something called Procfile on Heroku and add the command that should be executed after we deploy the code. Create that file at the toplevel of your project and insert:
web: npm run build && npm start
This tells Heroku to build the app using the app scripts (because there is already a step “build” inside your scripts section) and after that start our previously created server file which than hosts the www/ folder!
To push your changes and deploy the app to Heroku, simply run the following command whenever you make changes now:
ngit add .
git commit -am 'Heroku Dpeloyment.'
git push heroku master
After running the command Heroku prints the link to your website, or you can also run heroku open
to bring up your website. This page can now also be viewed with a mobile device and you get the platforms like in the image below.
Automation
If you want some more automation, you can simply put your ”deployment script” into one file. Therefore, create a new file and give it enough permissions:
touch herokuDeployment.sh
chmod 777 herokuDeployment.sh
Inside that file put everything you need after changing your apps code:
ngit add .
git commit -am 'HEROKU DEPLOYMENT'
git push heroku master
heroku open
This will commit your changes (and add files if you added something new), push everything to Heroku (where our build takes place) and finally open your website!
On your computer, the website in Chrome will then look like this:
Conclusion
It’s actually super simple to share your code base between web and mobile - your Ionic app is already a website!
By using Heroku we can easily deploy the full code of our app without maintaining a second repository and we are able to build the mobile app and the web app from one code base.
You can also find a video version of this article below!